I want to start by prefixing that this is a very messy approach that is not the easiest. There are use cases when you need this but there are simpler ways in some situations.
Easy Approach
For example, if you are simply interested to add new staff users but do not have the email functionality to confirm them - you can update invites table directly in database.
Step 1:
First create an invitation through admin, then go to database and update the status to sent. This will make sure that Ghost understands that email was sent (although it was not).
update invites set status = "sent";
Step 2:
Second, copy the token from database. You can fetch it with the below query. Copy it as you will need it in step 3.
select token from invites;
Step 3:
Go to a url in browser: https://<your-site-address>/ghost/#/signup/<token>. That is it, you can bypass the email part quite easily. (make sure to do this in a new session, because you cant be logged to sign up).
Once you get there you should be able to sign up with the new user.
However if you need something more then the below can be a good starting point to get somewhere. Read further at your own expense.
There are some cases where you might need to add an admin user to your Ghost blog from CLI. By default this is not possible and requires a custom script.
Unfortunately the custom CLI commands are fairly complex and there is virtually no documentation to add them. Since Ghost-CLI is separate code base it is difficult to hook into it, we may just need to wait for someone to break it down and describe it properly.
Thankfully there is a pretty simple way to create the user from CLI.
Let's get into it.
You will need SSH Access to your Ghost installation, file manager and ability to follow 4 steps.
Step 1: create the file
Create a directory current/core/server/commands/ and then a file in current/core/server/commands/add-admin-user.js.
// Load the Ghost environment
require('../../../ghost');
const UserModel = require('../../../core/server/models/user');
const { Command } = require('commander');
const program = new Command();
program
.command('add-admin-user')
.description('Add a new admin user')
.option('--email <email>', 'Email')
.option('--name <name>', 'Name')
.option('--password <password>', 'Password')
.action(async (options) => {
const { email, name, password } = options;
const adminRoleId = 1;
try {
await UserModel.User.add(
{
email,
name,
password
},
{}
);
console.log('Admin user added successfully.');
} catch (err) {
console.error('Error adding admin user:', err);
}
});
// Parse CLI arguments and run the command
program.parse(process.argv);
Step 2: Temporarily stop Ghost
We need to load the ghost environment in order to be able to execute the User model functions. There are cleaner ways to do this, but none as fast as just stopping Ghost and afterwards turning it back on.
Run: ghost stop
Step 3: Execute the script
First go to your Ghost installation root. Then you should execute the following command.
node current/core/server/commands/add-admin-user.js add-admin-user --email [email protected] --name TestUser --password atleast10chars
You need to pass the new user data to this command:
- password (should be at least 10 characters)
- name
In the terminal you should see the following message:
Admin user added successfully.
Since we required Ghost for environment purposes there will also be other messages. This is fine, to get out of this context simply press CTRL + C.
Step 4: Start Ghost and check that user exists
Since we stopped Ghost in step 2 we need to run it again. Run ghost start.
Then we can go admin ghost/settings/staff and see our new user.
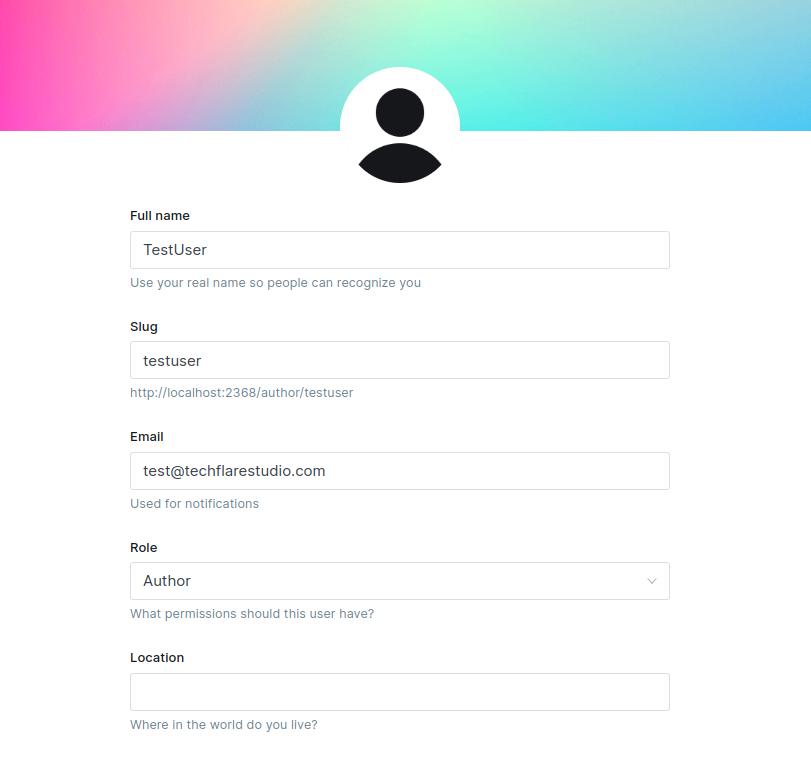
By default the user role will be Author, but you can change that in the script, or after it has been created in admin panel.
Final words
That is it. Overall it is quite simple to add the user, but definitively not as simple as it many other platforms. I hope that Ghost adds the documentation to create custom CLI commands which would make this much more clean and easy to do.