We will do this by writing a mixin that hooks into the shipping information save method. If you are unsure what a mixin is you may want to start by inspecting the default documentation. In short javascript mixin is a way to customize a javascript component without rewriting the component itself.
We can see that js/action/set-shipping-information.js is loaded in checkout. Lets start by inspecting if this is the correct component to hook into.
We can see that the component is simply using the Magento_Checkout/js/model/shipping-save-processor to save the shipping information to quote.
/**
* @api
*/
define([
'../model/quote',
'Magento_Checkout/js/model/shipping-save-processor'
], function (quote, shippingSaveProcessor) {
'use strict';
return function () {
return shippingSaveProcessor.saveShippingInformation(quote.shippingAddress().getType());
};
});
In order to hook into this first we need to declare a mixin. The mixins configuration in the requirejs-config.js
associates the original component with the mixin.
First you will need to create a module but I assume that part is already taken care of. Our module will be called Techflarestudio_CheckoutMixin. Once you have created a module you will need to create your requirejs-config.js file in view frontend area.
var config = {
config: {
mixins: {
'Magento_Checkout/js/action/set-shipping-information': {
'Techflarestudio_CheckoutMixin/js/action/set-shipping-information-mixin': true
}
}
}
};
As you can see we are creating a mixing for the action and defining our new action.
define([
'jquery',
'mage/utils/wrapper',
'Magento_Checkout/js/model/quote'
], function ($, wrapper, quote) {
'use strict';
return function (setShippingInformationAction) {
return wrapper.wrap(setShippingInformationAction, function (originalAction) {
/**
* Chance to modify shipping addres data
*/
var shippingAddressData = quote.shippingAddress();
/**
* Log before the original function
*/
console.log("Before");
console.log(shippingAddressData);
var result = originalAction();
/**
* Log after the original function
*/
console.log("After");
console.log(shippingAddressData);
return result;
});
};
});
Notice the usage of mage/utils/wrapper. This allows us to wrap the original function and execute actions before and after while still keeping the original function untouched. This allows the same function to be modified multiple times without rewriting or breaking the others adjustments.
That is it. Enable the module, compile the code and go to checkout. You should see the console logs in your browser after saving the shipping information.
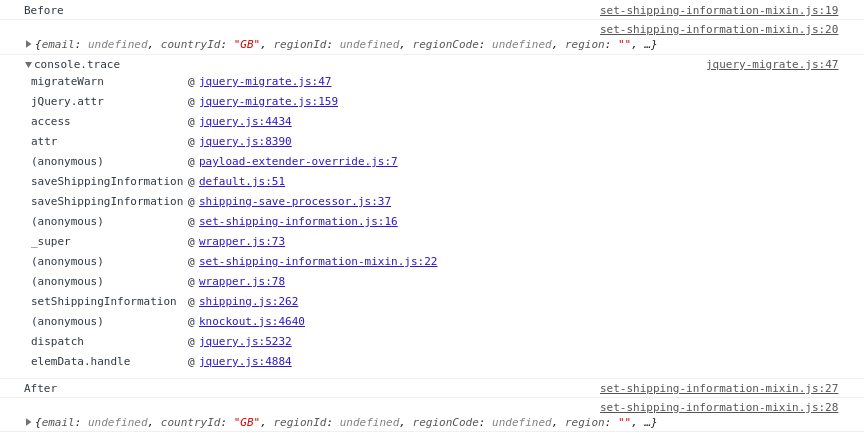