In this article we are going to explore AWS Lambda and ways we can utilize it for daily automation tasks. We will observe our CodeCommit repository and send Slack notifications for the events happening in the repository.
First you will need a AWS (Amazon web services) account. You can sign up for free tier account which includes most of the services and you can use them for free as long as you stay withing the defined limits - https://aws.amazon.com/free .
In our example we will be using a trigger coming from AWS CodeCommit. If you are unfamiliar with this service or would like to see how to set it up you can check this article https://techflarestudio.com/aws-codecommit/.
Creating the trigger
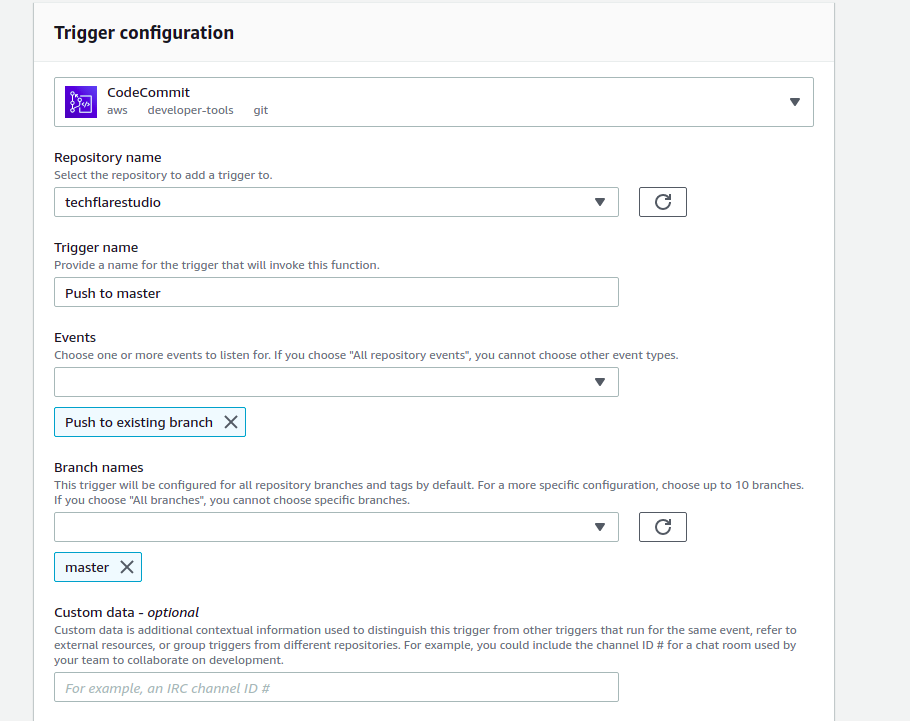
We will trigger the Lambda function when there is a push action to master branch in our Ghost repository for techflarestudio.
The goal for this observe the trigger and perform some type of action. In our example we will simply push a webhook to a Slack channel.
Create the function
By default the handler function is index.js but you can define any function you wish by editing the handler information. Paste this code into your handler function.
const request = require('request'); exports.handler = (event) => { request.post( 'https://hooks.slack.com/services/zzzzz/yyyyy/xxxxxxx', { json: { text: 'Techflare studio push to master' } }, (error, res, body) => { if (error) { console.error(error) return } console.log(`statusCode: ${res.statusCode}`) console.log(body) } ) };
As you can see we are using 'request' module to create the post request to our slack channel. By default 'request' is not part of the Lambda function so you need to upload the actual module yourself.
You can upload any node modules by using the upload by zip code entry point. Best way to handle this is to edit and test the code directly on your local IDE. Once it is ready you can zip the code and upload it. Make sure to upload all of the required modules (node_modules directory) as Lambda does not run npm install and requires you to provide all of the code necessary.
Here is how our function would look like in the AWS inline editor.
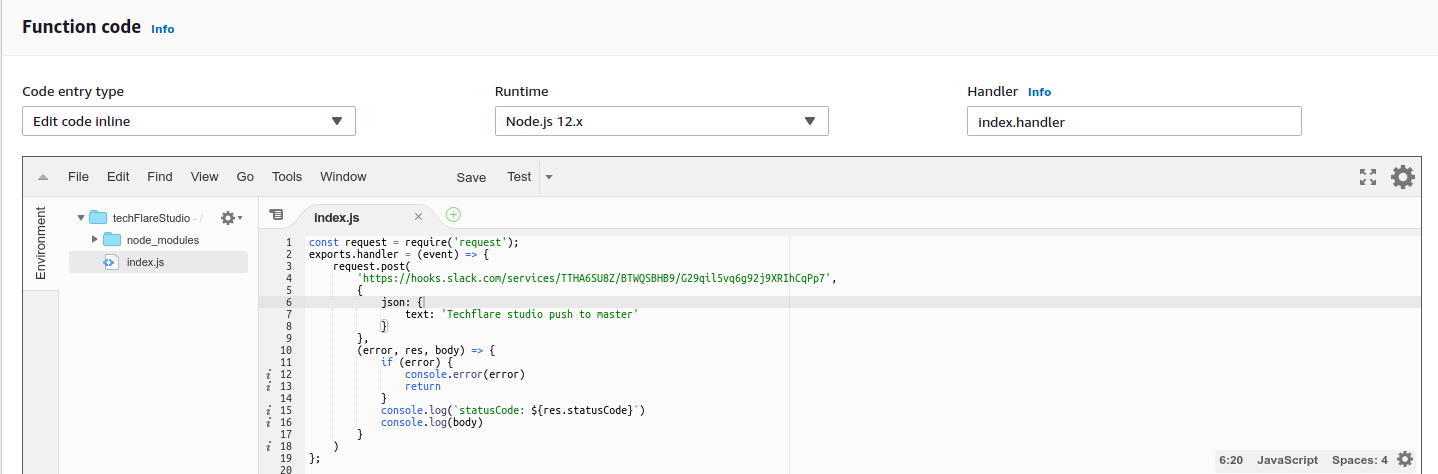
The actual Slack notification logic is self explanatory. Once the Lambda function is triggered we simply create a post request to the Slack webhook. We add the message in the "text" parameter. You could get creative here and include some event type variables in the Slack notification. For example, you could get the commit message or author. Perhaps even create some logic so that only some events trigger a notification. The possibilities are endless here.
You can test the event in the Lambda editor by triggering a custom event type. You can create a CodeCommit event type and test out receiving and passing out any data.
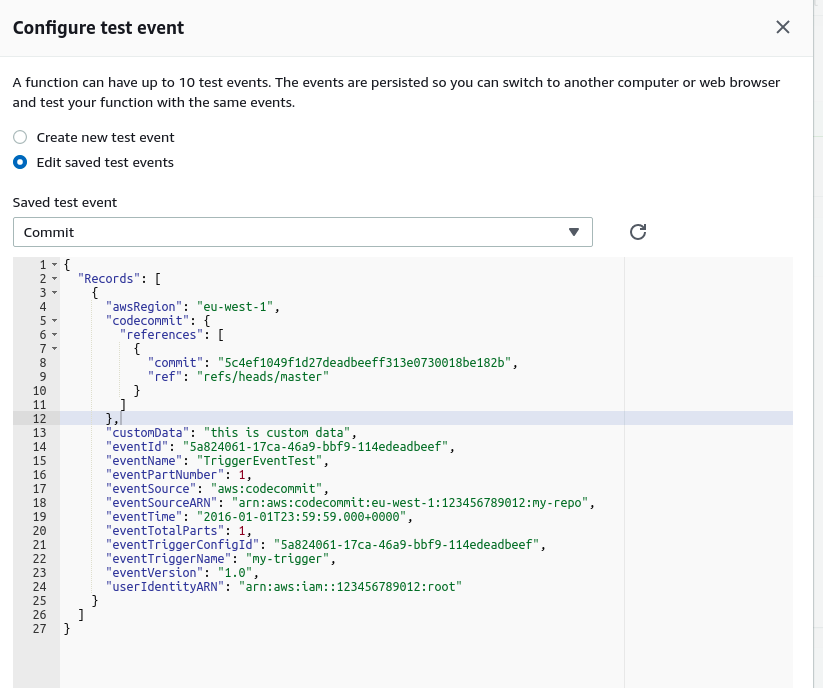
Once you have finalized testing and everything is ready you can save the function and test the actual implementation. In our example the trigger is push to master. So go ahead merge a new feature into master (according to your version control work flow). Once the changes are pushed you will receive a notification in your Slack channel that looks something like this:

Great. Now that the Lambda function is ready we can also see the metrics for the processing. There are charts that show error/success rate, how many function calls have occurred, duration of each call and much more. These can be extremely useful once we have a network of functions and top performance is a must.
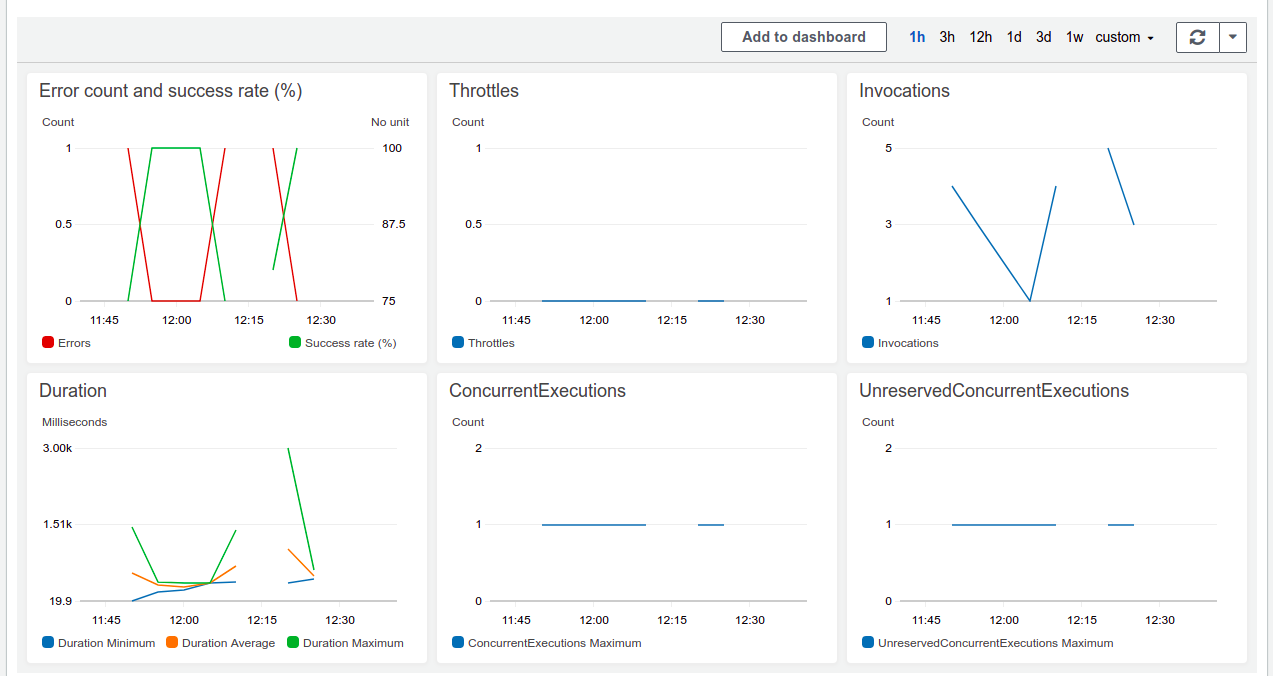
That is it for this article. We have created a very simply Lambda function which has a real life usage case. Hopefully this inspires you to create something for your own work flow. Let us know if you have any ideas or would like to see some deeper topics explored.
Our AWS exploration is just kicking off so make sure to follow us on Medium and Twitter.